1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118
//! Vkontakte API Rust client library //! //! [](https://gitter.im/kstep/vkrs) //! [](https://travis-ci.org/kstep/vkrs) //! [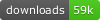](https://crates.io/crates/vkrs) //! [](https://crates.io/crates/vkrs) //! [](https://crates.io/crates/vkrs) //! [](https://coveralls.io/github/kstep/vkrs) //! [](http://issuestats.com/github/kstep/vkrs) //! [](http://issuestats.com/github/kstep/vkrs) //! //! [Documentation](http://kstep.me/vkrs/vkrs/index.html) //! //! # Usage //! //! Add to your Cargo.toml: //! //! ```toml //! [dependencies] //! vkrs = "0.5.0" //! ``` //! //! Then [add your app](https://vk.com/apps?act=manage) at [vk.com](https://vk.com/). //! //! Then authorize and use: //! //! ```rust,no_run //! extern crate vkrs; //! //! use std::{env, io}; //! use vkrs::*; //! //! fn main() { //! let api = api::Client::new(); //! let oauth = api.auth( //! env::var("VK_APP_ID").unwrap(), //! env::var("VK_APP_SECRET").unwrap()); //! //! let auth_uri = oauth.auth_uri(auth::Permission::Audio).unwrap(); //! // Or if you want to get permissions for specific request: //! // let auth_uri = oauth.auth_uri_for::<audio::Search>(); //! println!("Go to {} and enter code below...", auth_uri); //! //! let inp = io::stdin(); //! let code = { //! let mut buf = String::new(); //! inp.read_line(&mut buf).unwrap(); //! buf //! }; //! //! // You may want to save this token for future use to avoid asking user //! // to authorize the app on each run. //! let token = oauth.request_token(code.trim()).unwrap(); //! //! // The access token is JSON serializable with serde, so you can do it this way: //! // File::create(TOKEN_FILE).ok().map(|mut f| serde_json::to_writer(&mut f, &token).ok()).unwrap(); //! // //! // And then you can load it again: //! // let token: auth::AccessToken = File::open(TOKEN_FILE).ok().and_then(|mut f| serde_json::from_reader(&mut f).ok()).unwrap(); //! //! let songs = api.get(Some(&token), //! audio::Search::new() //! .q("Poets Of The Fall") //! .performer_only(true) //! .count(200)) //! .unwrap(); //! //! for song in &songs.items { //! println!("{:?}", song); //! } //! } //! ``` //! //! # License //! //! Licensed under either of //! //! * Apache License, Version 2.0 ([LICENSE-APACHE](LICENSE-APACHE) or http://www.apache.org/licenses/LICENSE-2.0) //! * MIT license ([LICENSE-MIT](LICENSE-MIT) or http://opensource.org/licenses/MIT) //! //! at your option. //! //! ## Contribution //! //! Unless you explicitly state otherwise, any contribution intentionally submitted //! for inclusion in the work by you, as defined in the Apache-2.0 license, shall be dual licensed as above, without any //! additional terms or conditions. #![cfg_attr(feature = "unstable", feature(custom_derive, plugin))] #![cfg_attr(feature = "unstable", plugin(serde_macros))] #![allow(unknown_lints)] #![allow(wrong_self_convention)] #![deny(unused_imports)] extern crate serde; extern crate serde_json; extern crate hyper; extern crate url; extern crate inth_oauth2 as oauth2; extern crate rustc_serialize; extern crate chrono; mod macros; pub mod api; pub mod auth; pub mod audio; pub mod gifts; pub mod photos; pub mod video; pub mod users; pub mod stats; pub mod status; pub mod wall; pub mod utils; pub mod execute; pub mod storage; pub mod account; pub mod notifications;